Note
Go to the end to download the full example code.
Line Plot Data Slicing#
Example showing data slicing with cosine, sine, sinc lines.
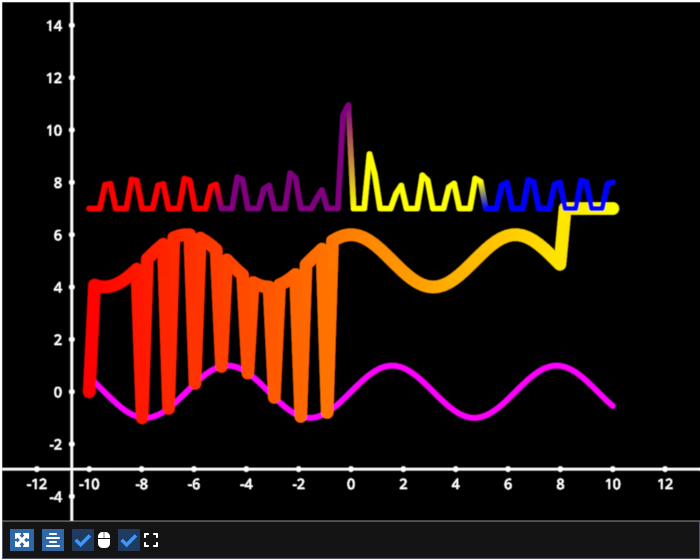
/home/runner/work/fastplotlib/fastplotlib/fastplotlib/graphics/features/_base.py:18: UserWarning: casting float64 array to float32
warn(f"casting {array.dtype} array to float32")
# test_example = true
import fastplotlib as fpl
import numpy as np
figure = fpl.Figure(size=(700, 560))
xs = np.linspace(-10, 10, 100)
# sine wave
ys = np.sin(xs)
sine = np.dstack([xs, ys])[0]
# cosine wave
ys = np.cos(xs) + 5
cosine = np.dstack([xs, ys])[0]
# sinc function
a = 0.5
ys = np.sinc(xs) * 3 + 8
sinc = np.dstack([xs, ys])[0]
sine_graphic = figure[0, 0].add_line(data=sine, thickness=5, colors="magenta")
# you can also use colormaps for lines!
cosine_graphic = figure[0, 0].add_line(data=cosine, thickness=12, cmap="autumn")
# or a list of colors for each datapoint
colors = ["r"] * 25 + ["purple"] * 25 + ["y"] * 25 + ["b"] * 25
sinc_graphic = figure[0, 0].add_line(data=sinc, thickness=5, colors=colors)
figure.show()
cosine_graphic.data[10:50:5, :2] = sine[10:50:5]
cosine_graphic.data[90:, 1] = 7
cosine_graphic.data[0] = np.array([[-10, 0, 0]])
# additional fancy indexing with boolean array
bool_key = [True, True, True, False, False] * 20
sinc_graphic.data[bool_key, 1] = 7 # y vals to 1
# NOTE: `if __name__ == "__main__"` is NOT how to use fastplotlib interactively
# please see our docs for using fastplotlib interactively in ipython and jupyter
if __name__ == "__main__":
print(__doc__)
fpl.loop.run()
Total running time of the script: (0 minutes 0.413 seconds)