Note
Go to the end to download the full example code.
Simple 3D Line Animation#
Example showing animation with 3D lines.
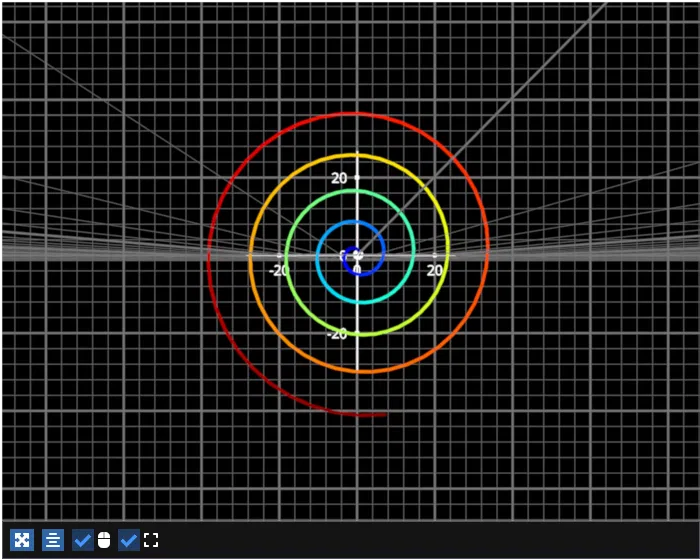
/opt/hostedtoolcache/Python/3.12.11/x64/lib/python3.12/site-packages/pygfx/objects/_ruler.py:272: RuntimeWarning: divide by zero encountered in divide
screen_full = (ndc_full[:, :2] / ndc_full[:, 3:4]) * half_canvas_size
/opt/hostedtoolcache/Python/3.12.11/x64/lib/python3.12/site-packages/pygfx/objects/_ruler.py:272: RuntimeWarning: invalid value encountered in divide
screen_full = (ndc_full[:, :2] / ndc_full[:, 3:4]) * half_canvas_size
/opt/hostedtoolcache/Python/3.12.11/x64/lib/python3.12/site-packages/pygfx/objects/_ruler.py:284: RuntimeWarning: invalid value encountered in divide
screen_sel = (ndc_sel[:, :2] / ndc_sel[:, 3:4]) * half_canvas_size
/home/runner/work/fastplotlib/fastplotlib/fastplotlib/graphics/features/_base.py:18: UserWarning: casting float64 array to float32
warn(f"casting {array.dtype} array to float32")
# test_example = false
import numpy as np
import fastplotlib as fpl
# create data in the shape of a spiral
phi = np.linspace(0, 30, 200)
xs = phi * np.cos(phi)
ys = phi * np.sin(phi)
zs = phi
# make data 3d, with shape [<n_vertices>, 3]
spiral = np.column_stack([xs, ys, zs])
figure = fpl.Figure(cameras="3d", size=(700, 560))
line = figure[0,0].add_line(data=spiral, thickness=3, cmap='jet')
marker = figure[0,0].add_scatter(data=spiral[0], sizes=10, name="marker")
marker_index = 0
# a function to move the ball along the spiral
def move_marker():
global marker_index
marker_index += 1
if marker_index == spiral.shape[0]:
marker_index = 0
for subplot in figure:
subplot["marker"].data = spiral[marker_index]
# add `move_marker` to the animations
figure.add_animations(move_marker)
# remove clutter
figure[0, 0].axes.grids.xy.visible = True
figure[0, 0].axes.grids.xz.visible = True
figure.show()
# NOTE: fpl.loop.run() should not be used for interactive sessions
# See the "JupyterLab and IPython" section in the user guide
if __name__ == "__main__":
print(__doc__)
fpl.loop.run()
Total running time of the script: (0 minutes 26.480 seconds)